Some simple editing tweaks are surprisingly useful. We create a macro to create a new paragraph with the current sentence.
Thanks for your interest
This content is part of a paid plan.
Create a new paragraph with a sentence
Create a new paragraph with the current sentence?
Sounds almost pointless at first … until you get used to using it. Such simple editing changes can be more useful than they may appear. It's like getting a gift you didn't know you wanted. I created this macro on a whim, but it's one of my favorites.
Create the empty macro
We're starting from scratch again, so a previous post covers creating an empty macro. The result is something like:
The single quote tells VBA the rest of the text on that line is a comment meant only for human readers, and we begin our macro steps on the empty line.
What are the manual steps?
If we create a new paragraph with the current sentence manually, what steps would we take?
- Move the insertion point to the beginning of the sentence.
- Delete the extra space between the sentences.
- Press return to create the new paragraph.
It’s just three steps but saving two steps every time add up over the course of a novel. Plus, it’s just nice not to have to do them.

Manual techniques are usually less efficient
Emulating the exact manual steps is often not the best way to write a macro in VBA, but we'll base this simpler version on it.
Goto start of sentence
The obvious Word VBA command we can use for the first step is the StartOf method:
StartOf collapses the selection and moves the insertion point to the beginning of ... what? This command works with a list of document Units. We want the current sentence, so we assigned the wdSentence constant to the Unit option with a colon equals := symbol.
StartOf has an important secondary effect of not moving beyond the beginning of the current sentence (or whatever unit).
Contrast with MoveLeft
If we were to naively use the standard MoveLeft method, the result is almost the same:
The MoveLeft method uses basically the same Unit step sizes. It might seem like a more natural command to use when just thinking about the manual steps, but it will move to the previous sentence if we’re already at the start of the current sentence.
StartOf stays at the beginning of the current sentence if we’re already there which is perfect for this macro.
Delete the extra space
At this point after the move command, the selection is just an insertion point (we purposefully did not use the Extend option with StartOf). Now delete the space between neighboring sentences using the Delete method.
Generally, Delete will do just that and remove the selected content from the document. If no content is selected, the command acts like we manually pressed the Delete key (it's not emulating the key, but the effect is similar). We need to delete backwards, so we assigned -1 to the Count parameter (with a comma separating the options). The default is to delete one character, but we need the negative value to delete backward in the document.
If you’re using Word for Mac, note the regular Delete command acts like a “forward" delete, deleting to the right in the document, as opposed to the typical "backward" delete action on Mac systems.
Insert the paragraph break
Enter the paragraph break using the InsertParagraph method.
Technically, this method replaces the current selection with a paragraph marker. If we want to be more specific, two sibling methods, InsertParagraphBefore and InsertParagraphAfter, allow us to insert a paragraph at the start or end of the current selection while keeping the current selection contents. Which one we use depends on the macro being written. Our selection is currently collapsed, so these all do practically the same thing.
Collapse the selection
The InsertParagraph command extends the Selection to include the new paragraph marker (which is a single character in Word), so we need to collapse the selection back down to an insertion point. The Collapse method does this for us:
But we need to collapse toward the side with the new paragraph, so we use the Direction option.
We assign the value wdCollapseEnd (from another Word constants table) to the Direction option using a colon equals := symbol. The insertion point is now positioned at the beginning of the new paragraph.
This step isn't quite how we would envision it when working manually. We would just tap an arrow key or something to cancel the selection. This is how we do that in VBA.
Finish the macro
Now we just put the commands together for our final simpler version of the macro.
Just a few easy-to-understand commands, but they perform a convenient action for us. I assigned my version to Option+Return in Word for Mac or Alt+Return on Windows. The extended content below solves a small issue with this simpler version emulating the manual steps.
Any gotchas?
You should get into the habit of thinking what might cause unexpected results or maybe even problems when you use your macros before rushing to implement your new productivity booster. I’m not expecting you to think like a programmer, but it doesn’t hurt to be a careful about special cases.
This is more of an issue for macros that delete or rearrange content. We definitely don’t want to lose a chunk of our work in progress because we didn’t think a little bit more when writing a macro. Today’s macro is less of a concern since we only delete one character in the document.
What are some special cases in this macro?
What if a starting selection exists?
A common special case to consider is whether the macro runs when text is already selected, intentionally or unintentionally. Sometimes our fingers just hit weird keys when we tap a shortcut.
A nice side-effect of the StartOf command up top is it automatically collapses the selection unless we explicitly set the Extend parameter. We don’t have to worry about whether the user starts with a selection or not because it collapses either way. This also makes the new paragraph step safer, so we don’t accidentally wipe out any text when we insert the paragraph. (The other insert paragraph commands will do so while keeping the initial selection contents intact.)
What about the last sentence of a paragraph?
If the insertion point is anywhere inside the last sentence (assuming it’s not the only sentence) of a paragraph, it will normally have a preceding space just like a “middle” sentence does. For this macro, there is no difference, so nothing bad should happen.
What about the first sentence of a paragraph?
Why would we run the macro on the first sentence of a paragraph? There’s no point in doing so because it already begins a new paragraph, but what if you hit the shortcut accidentally as you type? You meant to run another macro, but you tapped the shortcut for this macro. We should at least be aware of what would happen.
Our use of the Delete command above was intended to delete the preceding space separating two sentences, but it will also delete the preceding paragraph marker (or any other character present) if we run the macro when located inside the first sentence. Then the InsertParagraph step will recreate the paragraph.
Oh, okay. That seems fine … [ominous music plays in the background]
In fact, it will look like nothing happened most of the time, right?
Well ... about that.
Whether the macro should create an empty paragraph above the current when starting on the first sentence is a preference. The issue with this macro is a logical error when the previous character is a paragraph marker not a space.
If you want to know the details …
It's a minor side effect in this case (which is a good place to learn a lesson), but if you think a little more sequentially like VBA works, we could get an unintended consequence with the new paragraph's style.
If we’re creating a new paragraph from within or after the end of another, it will inherit that paragraph’s style. This is expected in regular typing since we expect a paragraph with the Normal paragraph style to keep producing paragraphs with the Normal style when we tap Return. (Some paragraph styles will automatically create a new paragraph with a different style, but that’s not happening in this macro.)
However, for the first sentence of a paragraph, our macro would first delete the paragraph marker and then replace it immediately with one of its own. Our macro would be joining the two paragraphs for just a moment before separating them again. As a result, the restored paragraph break will mysteriously inherit the style of the previous paragraph without anything else changing.
This isn’t a horrible side effect, but it might be a little aggravating six months from now after you’ve forgotten about it. “Why did that whole paragraph suddenly become a heading?”
First sentence correction
It’s almost like swatting at gnats, but for the perfectionists or hyper curious in the audience, let’s take care of this first-sentence error, so the macro doesn’t mysteriously cause paragraphs to change styles.
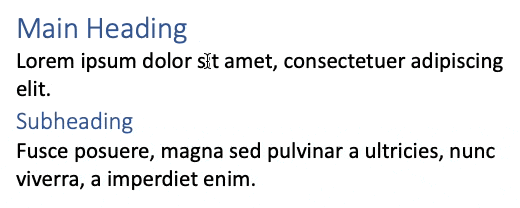
It’s almost a case of the treatment being worse than the ailment, but we can still learn something in the process.
How do we solve the issue?
We could work on a way to detect whether the insertion point is in the first sentence of the paragraph, but sometimes an easier solution is possible. Since the problem occurs only for one special case, we can reorder our steps to fix it. This fix is much simpler.
Avoid the problem by reordering the steps
The main problem occurs then the prior delete step deletes the previous paragraph marker rather than the intended space separating two sentences, so let's insert the paragraph before the delete step. Here is a revised order:
- Move the insertion point to the start of the current sentence just like before
- Insert the new paragraph
- Collapse the selection
- Delete the preceding space or paragraph marker
- Move to the beginning of the new paragraph
This isn't exactly thinking like VBA, but the improved command order isn't obvious either. We needed to consider the effects of each command on the result and pick a sequence that worked for ever regular and every special case.
Now the delete step will delete either the preceding space or the new paragraph marker. This is the crus of how we sidestep the first paragraph problem in the version above. Seems simple enough.
So, we reordered the steps, but we added a extra step at the end. Why?
Testing for one reason. Carrying out the steps, we would find what we're ending with the insertion point at the end of the prior paragraph.
Let's work back through the revised steps.
Move to the start of the current sentence
Step 1 is exactly the same as above and still occurs first.
The insertion point is now guaranteed to be located at the beginning of the current sentence.
Insert the new paragraph
In step 2, we insert the paragraph at the insertion point. This can still be done with the same InsertParagraph method as above just done one step earlier.
Don't forget this particular command replaces the selection which is fine for our macro since the "selection" is just an insertion point. If you want to preserve the selection (in another macro) and just add a paragraph to it, use InsertParagraphBefore or InsertParagraphAfter instead.
After this command, the selection spans a single paragraph marker.
Collapse the selection
The Selection will extend to include the new paragraph. We did this step above, but this time we should use the Collapse method to collapse toward the beginning of the selection.
Delete the space or paragraph marker
At this point, a space usually resides on the left side of the insertion point and our new paragraph marker on the right. For the original first sentence of a paragraph, the difference is another paragraph marker will be on the left side instead of a space. Either way, we want to delete the character to the left with the Delete method.
As we did above, we assigned a negative Count value to delete backward in the document.
Move to the new paragraph
Now the insertion point should be at the end of the previous paragraph, but we want to finish the macro with it positioned at the new paragraph. The best choice is to move just one character forward past the paragraph marker into the new paragraph. The easiest way to do this is with the MoveRight method.
The default move is one character forward in the document which is exactly what we need.
Alternative move method (not used)
An alternative would be the MoveDown method, which moves down by one line by default.
While this works, it will position the insertion point somewhere inside the sentence, but we will not have control over where because it depends on the length of the last line of the previous paragraph. It would have the effect of moving your insertion point to a "random" location in the new paragraph sentence which is awkward.
The suggested changes in the member version avoid this quirk entirely with a more intuitive default behavior for the macro.
Final macro with first sentence fix
That’s a lot of talking for a small issue, but the fixed version handling all paragraph sentences correctly is:
Such a simple solution will not always work out, but it's nice to see here. It's a good example of not having to get more complicated to solve a problem. It's a slight improvement overall, but it's better when your macros don't have little quirks that can become annoying over time.
Comment on comments
Notice how I explained the problem and reason for the command order. Such comments will help if you ever come back to your macro since the reason for this particular order isn’t obvious.
I had this happen to me while rewriting my own version of this macro. I ignored a poorly worded note and initially tried to remove what seemed like several superfluous steps, but the gotcha turned out to be real. While I did waste some time by ignoring my own comment, I would have wasted even more time re-solving the same issue if I had not explained it to my future (now present) self as a comment.
Improvements
The above macro is fully functional, but what can we do better? A follow up member version will solve these (small) issues.
Avoid screen jitter
When used on the first sentence, the above macros may cause the sentence to jump up and down, so it would be nice to use a different approach to avoid this unsightly behavior.
Fix insertion point side effect
When used on a first sentence, the insertion point jumps to the beginning of the sentence. It's an odd side effect when no new paragraph is created.
Catch extra spaces
I like my macros to automatically catch odd spacing issues when running. For example, if more than one space separates the sentences, why not let the macro go ahead and fix that on the fly.
Unfortunately, another issue creeps into the mix if we want to catch and correct for these extra spaces between sentences. We basically need to thumb wrestle Word into submission because Word often likes to put one space back because it thinks we're making a mistake.
Do not move the insertion point
You may like the insertion point finishing macro at the beginning of the new sentence, but I prefer it remains wherever I was already typing.