Automatically selecting all text in nearby dialog or parentheses happens enough that writers can benefit from a faster way, so we create a macro to accomplish the task.
Thanks for your interest
This content is part of a paid plan.
Select dialog or parenthetical text
In the category of alleviating tedium from everyday writing and editing tasks, we create a macro that selects dialog or parenthetical text. Selecting specific chunks of a text is a common task, and dialog or parenthetical text make good candidates for a set of targeted selection macros.

I use the parentheses versions more in my work documents, but the differences between the two macros are almost trivial. The most practical implementations need one macro to search forward or backward in the document for each set of punctuation marks (see below for why). The member version includes additional checks to prevent any spurious selections (more annoying than a problem) when the punctuation does not exist in the document search direction.
Double quotes in VBA
We need to know how to specify double quotes in VBA. The problem is a plain text string is defined in VBA with double quotes like "some text." How can we include double quotes when double quotes are used to specify the string?
Just for clarity, these are the straight double quotes that our keyboard types. Word automatically changes them in the application to left or right curly double quotes which Word calls "smart quotes."
Just say no to VBA double quotes notation
VBA allows a clumsy notation of an empty pair of double quotes inside some double quotes """some text.""" Each pair of double quote inside the outside double quotes is a single double quote in the plain text string. Clear as mud?
Ughhh … VBA has been around for a while, but are we neanderthals or humans?
We might as well go hunt mastodons for supper if we use that notation. While it takes a few extra steps, it's clearer if we just define our own double quote characters. The easiest way is to assign the characters to variables. Using constants are even better since the characters don't change, but we'll omit that to avoid the extra explanation.
Represent double quote characters in VBA
VBA includes a standard function ChrW(…) that allows us to specify special characters. Often, such characters are already used for other things in VBA like the straight double quote character or a paragraph mark. Others may correspond to characters our keyboard does not include like left or right curly double quotes.
A small number of characters are defined in a (mostly) standard ascii table which was defined in the early 1960s, but that table doesn't include the left and right double quotes. We need a larger Unicode character table that includes almost every concrete symbol in the world (almost 155 000 characters in its latest update).
Look up the character we need and cross-reference its assigned number. Then we use that number in the character function ChrW(…). The respective numbers for the quote characters are:
- Straight double quote " → ChrW(34) [not used in the macros below]
- Left double quote “ → ChrW(8220)
- Right double quote ” → ChrW(8221)
In VBA, we simply assign the respective character to a more convenient variable name.
These assignments are a little clumsy since we would need to define them in every macro where they are used, so a better approach is to define them as constant values for all macros. Either way, we can then refer to the respective variable or constant name when we need the character.
In macros dealing with dialog, we usually need to consider all three characters since Word may be prevented from converting a straight double quote (the keyboard one) to one of the curly double quotes. In this macro, left and right indicate the respective dialog boundaries, so we'll ignore the straight double quote.
Create the empty macro
A previous post covers creating an empty macro like the one shown below. The result is something like:
The single quote tells VBA the rest of the text on that line is a comment meant for human readers. We start our macro steps on the empty line.
For the best implementations, we'll need a second macro to search forward in the document and two more to select parentheses in either direction. We'll work with the backward dialog version for clarity, but the changes at the end for the other three macros are simple.
How will the macro work?
Before we create the macro, we need to decide what we want it to do. What result do we want? What will it search for in the document?
It sounds obvious, but do we assume the writer runs the macro when the insertion point (i.e., the blinking I-bar) is already between the quotes? Or do we want our macro to find nearby dialog elsewhere in the text?
The former is more natural and a tad simpler to implement due to the assumption of starting between the double quotes, but the latter is more useful in practice. The differences are small, but the specific steps matter if we want a specific result.
A macro is more practical, in general, when we don’t have to remember specifics about how to use it correctly. It just works. The trade off is we need one version to search backward and another to search forward in the document. Both versions work the same if the insertion point happens to already be between the double quotes (or parentheses).
Rough macro steps
What are the steps from a bird's viewpoint?
- Move backward in the document to a left double quote
- Select the left double quote
- Select over to the right double quote
- Select the right double quote
- Select any trailing spaces (like Word does with its automatic selections)
Then we're done. The character selections are may not be immediately obvious, but we need to include them in the selection somehow. VBA has several commands that make this sequence of steps almost easy.
As we develop the macro below, we’ll work on the backward version and tweak it to search right in the document and then to search for parentheses instead. The changes are minor.
Assume smart double quotes
A small logical problem arises casting a shadow over our macro aspirations.
I usually try to be general, but in this macro, a straight double quote has no direction associated with the character like the other double quotes do. If we try to account for straight double quotes in the logic, we won't know which side of the dialog we're on when we find the character.
How would the macro “know” that a straight double quote is at the beginning or the ending of a bit of dialog? It’s possible to work it out in the macro, but the extra cases and steps necessary to handle it quickly get messy. Even worse, we gain almost nothing in terms of a functionality boost, so it's not worth the time and effort for the few occasions we'll use it.
With this in mind, we'll simplify the macros by assuming smart quotes are turned on (in AutoCorrect options), so the document (almost) always uses left and right double quotes around dialog. This significantly reduces complications with interpreting the beginning and ending of dialog compared to when straight double quotes are allowed.
Find the beginning of some previous dialog
A previous macro allowed us to quickly navigate to various punctuation marks in a document. We'll use some of the same commands in this macro to achieve a different effect.
We want to find a set of double quotes anywhere in the document before the current insertion point. We start by searching backward in the document for a left double quote which we called LeftDQ (see why it was helpful to define those constants earlier?). This step is quite general since the insertion point doesn’t need to be between double quotes to work properly.
Move to a left double quote
The command to move the Selection to a specific character is the MoveUntil method.
The dot . notation allows us to reference a method (an action) or property (some data) of the Selection in this command.
The command automatically collapses any initial selection and moves the insertion point until it finds a certain character (or any of several characters, in general). The character set is specified as plain text, usually in double quotes like "abc", by assigning it to an option named Cset. At this point in the macro, we're only looking for a left double quote character.
The option assignment requires a colon equals := symbol. In this example, we're only searching for a single character which we called LeftDQ.
Since we're searching backward in the document, we also need the Count option.
It may seem awkward to use the Count option to search backward, but it tells the command how many characters to check until it finds a match with positive values indicating forward searches and negative values indicating backward searches. The Word constant wdBackward is a large negative value (from a small Word constants table) meaning the command should move backward in the document by as many characters as necessary to find a match. The two options must be separated by a comma.
No selection exists yet
Once the quote is located by the command, the insertion point is moved just to the right of the character. At this point in the macro, the Start and End positions of the Selection are at the same position in the document. Since these positions are the same, no content is spanned in the document yet.
See the gotchas below about possible spurious selections if the left double quote doesn't exist in the document anywhere before the insertion point.
Select the dialog text
Now, we extend the selection across the dialog text, but it takes a few steps to get everything.
Select the left double quote
At this point in the macro, the Start position of the Selection is positioned immediately after the left double quote. We probably want to include the quotes as part of the selection, so we use the MoveStart method to extend the Start position backward over the quote.
MoveStart tells Word to move the Start position of the Selection by the given Unit type, but we omit the Unit option since the default is to move by a character which is what we want.
Unfortunately, the default is to move forward, so we must use the Count option to specify a backward move.
Unlike MoveUntil command above, the Count option with MoveStart moves it by a specific number of units. We specify -1 to tell the command to move backward (the negative) by 1 Unit which includes the quote character in the selection.
We finally have something selected
Huzzah!
The End position of the selection was not changed with this command. Since Start and End now have different positions in the document, we have a real, albeit incomplete, selection. Yay for progress!
Find the right double quote
Since we found a left double quote, we are (somewhat) confident (or hopeful?) our incomplete selection is currently at the beginning of a pair of the left and right double quotes. We can now select the rest of the dialog text.
How?
We move the End position of the Selection over to the right double quote on the other side. We don’t change the Start position for the rest of the macro.
Extend the selection over the dialog text
The MoveEndUntil method moves the Selection’s End position to a specific character (or any of a set of characters).
Like the MoveUntil method above, this command requires a character set assigned to a Cset option, but it only moves the End of the Selection. We are looking for a right double quote which we assigned to a variable named RightDQ earlier.
The default direction is forward, so we can omit the Count option. The Start position is unchanged by this command, so it extends the End of the Selection forward in the document to span most of the dialog text.
Include the right double quote
At this point in the macro, the End position of the Selection is just before the right double quote. Most of the time, we’ll want to include it as part of the selection, so we use the MoveEnd method.
We don’t need any options with this command because the default Unit is to move by characters, and the default Count is to move by one Unit forward.
Include any trailing spaces
Including any spaces on the right side of the selection mimics how Word usually works with automatic selections such as when Word spans a whole word for us. Unless we have a good reason, it's a good practice to keep our macros consistent with how Word works.
The appropriate command to extend the selection over any trailing spaces is the MoveEndWhile method.
Similar to the MoveEndUntil command above, MoveEndWhile needs a character set to try to match them in the document. We again assign the desired characters as a plain text string to a Cset command option.
The difference is the MoveEndWhile command moves the End position of the Selection while it keeps finding any of the specified characters in the set immediately at the End position. At this point in the macro, we’re just extending the selection over any trailing spaces, so we assign a single space " " to the character set option.
The default is to extend the End of the selection forward in the document which is what we need here, so we can omit the Count option. The Start position of the selection is unchanged by this command.
Modifications for searching forward
The changes to search forward in the document are relatively obvious, but let's work through them at a quicker pace for completeness.
Initial forward move
We begin by moving forward with the MoveUntil method again, but the options are different.
We're searching for the right side of the dialog, so the command moves until its find a right double quote character which we called RightDQ. The default direction is forward by any number of characters until we find a match, so we can omit the Count option.
Select the double quote
We move the End position of the Selection forward by one character over the right double quote using the MoveEnd method.
No options are necessary since the default behavior is to move the End position by one character forward which is what we want.
Extend over any trailing spaces
To include the trailing spaces (like Word does by default), we also move the End position over any trailing space characters using the MoveEndWhile method.
Technically, this extension could be done anywhere after the initial move since the End position isn't changed again, but it fits better here with the forward movement and quote selection.
Backward selection
We now extend the Selection backward by moving the Start position until it finds a left double quote which we called LeftDQ. The command is the MoveStartUntil method.
The Count option for backward is required because the default is to move forward.
Extend over the left double quote
Finally, we include the left double quote in the selection using the MoveStart method.
We need to move backward, so the Count option is set to -1 meaning move backward one character which is the default move unit.
Gotchas
What could go wrong? We don't have to think like Mr. Murphy, but we should at least be careful.
Does an initial selection matter?
Does it matter if the user runs the macro with a selection already made in the document?
The initial selection mostly doesn’t matter in this macro because we start by moving the insertion point to a left double quote. Most move commands automatically collapse the Selection for the move (some move commands allow an optional Extend parameter).
This could affect the final selection in certain obscure cases since the move command works from the “active” side of the Selection, which is usually the Start of the selection, but the distinction will rarely affect everyday usage.
Mixed smart or straight double quotes
Occasionally, Word will not properly apply the left or right smart quotes which may leave one or the other as a straight double quote as typed by the keyboard. Since the macro uses the smart quotes to detect the extent of the dialog, the macro will select the wrong text. It's an infrequent issue that takes a lot of effort to catch, so it’s not worth the effort to rework the macro to account for it.
Do the double quotes exist?
We assumed the pair of double quotes existed in the document in the desired search direction. Do they? Is it a problem if they don't? It's useful to understand some of the details about what could happen.
Does a left double quote exist?
When the macro is run, it begins the selection by searching for a left double quote anywhere before the starting position. What happens if it doesn't exist?
The MoveUntil command simply does not move the Selection if no character is found.
Is that bad?
It's a tad problematic given the intention of the macro, but it's more of a quirk since we would just end up with a selection that is not bounded on the left side by a left double quote. If either double quote is missing, the macro will likely just create a spurious selection. The discrepancy would be immediately visible on screen, so the writer would then presumably recognize the mistake and ignore the selection.
Do I like it?
No, but is it worth fixing?
Also probably no since it increases the number of steps and complicates the macro all for a limited benefit.
Selection side note
This issue is an example of why manipulating the Selection directly is not ideal in more complex macros. If a problem is encountered later in the macro, previous changes have already been made to the Selection, so we're stuck somewhere in the middle of the process. The member version tackles the same task more easily using a Range variable, so it can validate the result before selecting the identified dialog range.
Final macros
Putting all of the above steps together, the final macros are below. Neither requires the user to run the macro with the insertion point between the pair of double quotes.
Select double quotes backward macro
To select all text between the previous set of double quotes in the document, the macro is:
The extra comments at the beginning of the macro are not required, but this macro has several point or issues that should to be mentioned for clarity.
In Word for Windows, I assign this to Alt+Shift+Single quote.
Word for Mac (really just MacOS, in general) has problems reading some shortcuts when modifier keys are combined with the punctuation marks. I prefer to assign this macro to something that makes sense like Command+Shift+Single quote or maybe as a second choice Command+Control+Shift+Single quote. Unfortunately, Word for Mac will not recognize these key combinations. I settled for Option+Control+Single quote which is a different shortcut pattern than most of my other selection shortcuts since it doesn’t include the Shift key.
Select double quotes forward macro
The version to search forward in the document is:
I assign this to Control+Shift+Single quote in Windows and Command+Control+Single quote in Word for Mac.
Why are there two macros?
For anyone who skipped the above descriptions, we designed the macro to find dialog text even if we do not start with the insertion point between the double quotes. This behavior is more useful in practice, but it means we need two slightly different versions. The little bit of extra work is worth the effort.
Adapt the macros for parentheses
Parentheses appear often enough in notes and work documents that it's nice to emulate the above macros to work with parenthetical text.
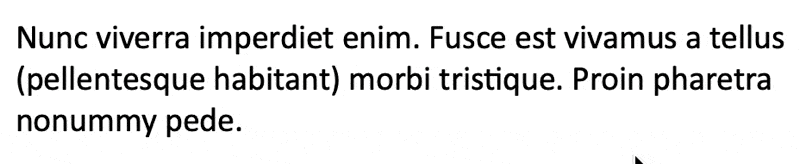
The conversion is nearly trivial. We mostly just swap out the left double quote for an open parenthesis and a right double quote for a close parenthesis. In the macro move commands described above, literally change the two quote characters to the corresponding plain text parenthesis in each macro.
- LeftDQ → "("
- RightDQ → ")"
Copy the macros and give them unique macro names. Make the two character changes in each macro. Delete the double quote variable declarations since they are not needed in this version. Of course, edit the comments to refer to parentheses instead.
Final selection parentheses macros
Making the above changes, the final versions to select parenthetical text are included below for completeness.
Select parenthetical text backward macro
To select all text between the previous set of parentheses in the document, use:
I assigned my version to a Command+Shift+9 shortcut in Word for Mac and Control+Shift+9 in Windows.
Select parenthetical text forward macro
To select all text between the next set of parentheses in the document, use:
I assigned my version to the shortcuts Command+Shift+0 in Word for Mac and Control+Shift+0 in Windows.
Improvements
What could we do better?
Use a Range variable
The member version uses a Range variable for the movement and extensions. While the end result is the same, the range allows us to only present the final result where the Selection updates the screen in real time (unless we turn off screen updates) as changes are made. For another plus, a range variable allows the macro to more easily validate the result and only select it when finished if the dialog range exists in the document.
Select the full sentence?
One of the things I like about VBA in Word is we can tweak our macros to work the way we prefer for our own workflow. You may prefer to select the full sentence including any dialog tags along with the quoted text.
I like the idea. On the other hand, a separate macro already selects a whole sentence, but this improvement would still be useful if the dialog contains multiple sentences. It only take a single extra line, but it's outside the scope—[… can't resiiist]:
Not a lot of explanation for brevity, but place the command at the end of the macro if you like this variation. It causes no additional problems if the dialog already spans a full sentence since the expansion simply does nothing in that case.
Use an undo record?
Often, when a macro contains multiple smaller changes, it's a good candidate to use an undo record. Meaning, the macro tells Word to package all the changes into a single undo step. They're convenient and make our macros feel more professional. However in these macros, we can get all the benefits by just using a range variable and adding a validation step before selecting the final result. This approach avoids the issues that can pop up with undo records.
Use functions?
Given the similarity in the macros, they seem to asking to be reimplemented as functions. Then we would create two worker macros that simply call those functions. While it is annoying and clunky to have all the repeated steps, this is a case where these two sets of macros are the only two practical applications.
We're only looking for a single character in either direction, so what else would we do?
- We could add square brackets, but that will be rarely used compared to parentheses. Seems more like a waste of time.
- Commas are a potential practical extension, but they would need to be implemented differently because they could cause logical problems. No direction is implied with commas in general text. They could even be in different sentences, so we would need some extra sentence detection logic … so it needs a separate macro.
My suggestion is to only implement them as functions once you're very comfortable with the process on macros that have more repetition (such as novel note macros). At that point, the improved organizational aspect is more agreeable even with the increased complexity the functions bring.