Toggle the style of the current paragraph between two common styles.
I use these style toggles regularly in my novels when outlining and organizing content as I write.
Thanks for your interest
This content is part of a paid plan.
Create the empty macro
A previous post covers creating an empty macro like the one shown below. When you’re done, you’ll have something like:
The single quote tells VBA the rest of the text on that line is a comment meant for human readers. We start our macro steps on the empty line.
What is it?
The idea is to tap the keyboard shortcut once to change the style to the default one and twice if you want the second style. It’s very fast since your fingers are already there on the shortcut keys.
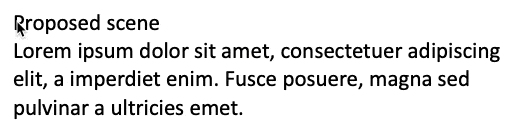
Basically, instead of having to remember a separate keyboard shortcut for each common paragraph style, combine similar styles into a single keyboard shortcut and let the macro decide which one to apply.
Huh? Why?
I like to keep my fingers on the keyboard as much as possible. I particularly dislike hunting through the Quick Styles panel or the Style Pane.
It’s painfully slow even for the Quick Styles panel (to me at least).
I eventually realized there are standard keyboard shortcuts to apply the Normal paragraph style or heading styles, but even that isn't helpful since I have a stack of related custom styles I use for my novels as write.
How I use style toggles
For clarity, here is a concrete but abbreviated example of how I use the style toggles.
I outline as I write using paragraph styles, including scene and subscene headings like:
- Scene Idea – A planned scene likely to be used as I progress in the document. Usually I’ll have scene notes about important elements or snippets for when I write the actual text.
- Scene Tentative – A tentative scene with some elements I like but not necessarily included in the final story.
- Scene Heading – Scene heading style for scenes where I have completed the rough text.
There’s more (like scene forks, edited content, notes, etc.), but this gets the basic idea across.
This approach helps me keep my ideas and progress organized during both the planning and writing phases (they overlap a lot for me).
Basically, I combine two or three styles to a single keyboard shortcut , so I don't have as many combinations to remember.
It may be particular to my own writing process, but I thought you might like the idea.
Conditional Refresher
We’ll need conditions to toggle the paragraph styles. I’ve introduced them a couple times in the past, but in case you missed it, here is a refresher.
Condition checks (If Then Else statements)
An If Then Else allows us to check a condition and make a decision in our macro about whether to run certain steps if the condition is true or others if it is false.
It does the first part if the condition is true and the Else part if the condition is false. The else part with its steps is optional, in general.
How do we check a style?
Let’s build the steps of the macro.
Get the current paragraph
We’ve been using the Selection a lot to get the current paragraph.
Remember paragraphs are “things” (objects) with properties and actions that they can carry out on their content.
We’ve used this before for things like deleting the first paragraph (where we used the Range of the paragraph).
Here we want the paragraph itself, not the range it spans in the document text. Specifically, we want the paragraph’s style property.
Since we’ll be dealing with an insertion point rather than a range of selected text most of the time (see first gotcha below), we can simplify this using a shorthand:
Comparing styles
Now we need a way to check the paragraph style. We can check directly like so:
This would be our SomeCondition for the condition check above.
It may be a little confusing, but this also happens to be the way we set a new style for the current paragraph to the style called Style Name.
In either case, we have to put the name of the style in double quotes.
Putting it into a conditional If Then Else, we get:
Toggle the paragraph styles
Now we finally get to the toggle part of the macro.
Basically, if the current paragraph is already a Scene Idea paragraph style, we want to automatically make it a Scene Tentative style.
Otherwise default to setting the paragraph style to the Scene Idea style since that is the more common case (at least in my own writing when I’m starting a new novel).
The Selection.Style = “Scene Idea” in the If Then checks whether the style is as indicated (which must be true or false).
When Selection.Style = “Scene Idea” is used below on a separate line, it sets the style of the Selection paragraph.
These assignments don’t have to be static either. You can change them as you get to different stages of your novel.
Any gotchas?
We do have a couple issues to worry about for this macro.
Starting selection
If the user runs the macro with a selection already made that spans more than one paragraph, we want to avoid any potential problems. The easiest way to handle this case is to just collapse the Selection before we do anything else.
Now we know we’re working with an insertion point for the rest of the macro steps.
Invalid styles
The more frustrating gotcha occurs when the paragraph style doesn’t exist in the current document. It will crash the macro on the offending style assignment.
You might think it wouldn’t happen, but what if you create a new document and the style isn’t there (i.e., it isn’t part of your Normal template)?
Of course, we can take steps to ensure our styles are consistent across our works in progress, but it’s going to happen. Sometime.
To my knowledge, it can’t corrupt the document or delete any novel text, which is somewhat comforting as a writer, but it is still aggravating when your macro crashes and you get a popup window complaining at you.
VBA should at least allow us a better way to catch the error and let the macro end nicely, but it doesn’t, so we have to work around it.
Unfortunately, we don’t have the machinery to work around it right now. I’ll introduce a separate macro later explaining how to check whether a style is valid or not before you try to assign it to a paragraph. It’s a little complicated.
Finish the macro
So the final macro is:
I assigned this macro to Command+Control+H in Word for Mac and Control+H in Windows.
By the way, I regularly override default keyboard shortcuts that Word provides since I’d rather have my favorite commands at my fingertips than a few barely used ones.
Of course, you can create your own variations for any combination of styles. I have several combinations.
Cycle between three or more styles
There are two ways to add a triplet condition. We’ll stick with the easier version that is consistent with what we know so far.
Condition checks (If Then ElseIf Else statements)
As previously mentioned, If Then Else allows us to check a condition and make a decision in our macro about whether to run certain steps if the condition is true or others if it is false. We can extend this idea by adding an extra ElseIf condition check before the final Else.
As with a regular If Then Else, the Else part is optional.
Don’t tell anyone, but you can actually chain more ElseIf Then conditions together, but it gets awkward. There is another command, Select Case, that helps when you have many conditions, but three is a good limit for our style cycle macro, so we’ll stop there.
Finish the macro
Now we just put the commands together.
I assigned this macro to Command+Option+H in Word for Mac and Control+H in Windows.
Improvement
I have tried as many as four styles within the cycle, but that’s too many in my experience.
I’ve also added some steps in a few cases to automatically add the style if it isn’t found, but that is a topic for another day.