Create a new paragraph at the end of the current heading.
This one is nice one for novel notes where you regularly append ideas to the end of a heading and work in progress outlines (I outline as I write).
Thanks for your interest
This content is part of a paid plan.
Insert New Empty Paragraph
Huh? Isn't that what the Return key is for?
I'm talking about streamlining the process.
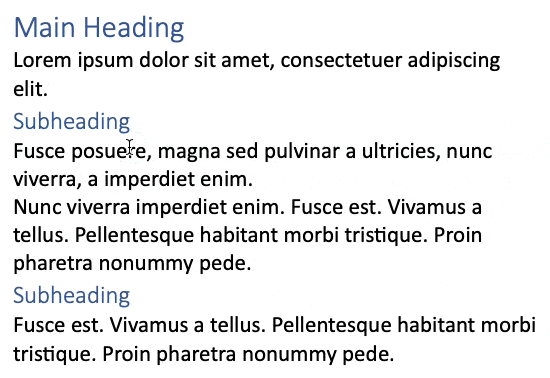
The idea is to create the paragraph at the end regardless of where you are in the heading content.
Create the empty macro
A previous post covers creating an empty macro like the one shown below. When you’re done, you’ll have something like:
The single quote tells VBA the rest of the text on that line is a comment meant for human readers. We start our macro steps on the empty line.
New paragraph below current heading
There are two main ways to accomplish this. We’ll take the easier way first followed by the slightly more involved method in the extended content below.
New paragraph below a standard heading
This version applies to standard Word heading styles Heading 1 – 9.
First we goto the next heading as mentioned in a previous article.
Now we find ourselves at the heading just below where we want our new paragraph.
Call a previous macro to finish
Now we can “call” an old macro we previously created to insert a new paragraph above the current heading paragraph (see the original article for the previous macro).
That's it. We're done.
What. Did. We just do?
Did you feel the electricity crackle through the air?
We just used a macro within a macro.
Given our position based on the initial heading jump, we took advantage of another macro without having to reinvent the wheel to get it done.
Just include both macros (placement doesn't matter), and the old one should automatically be used when the main macro today calls it.
Any gotchas?
What happens if we run the macro at the end of the document? (Just trying to give everyone ideas of what potential problems to consider.)
Turns out the macro doesn't work since there is no heading below the current one to jump to, but the extended version below solves this small issue as a side effect.
Finish the macro
Now we just put the two commands together.
I assigned this one to Command+Control+Option+Return in Word for Mac.
As previously mentioned, the above version doesn’t work for derived headings, but most of my novel notes use the standard heading styles Heading 1 – 9, so I could probably get by with this for many of my use cases.
A more general version will be in the members’ content at some point since a full implementation is a little more complicated than you might initially think, but the extended content below covers some of the improvements.
Improvements
This version for derived headings takes a different direction.
Add new paragraph at the end of a heading
As discussed in the extended content of the Goto Heading article, if we want to goto an arbitrary heading paragraph while allowing for derived heading paragraph styles, we have to use a different method than the stock Selection.Goto command.
Get the heading Range
We first need to get the Range of the heading, so we can target its end position for our new paragraph. As before, we take advantage of the hidden Word Bookmark for the current heading content.
A Bookmark is basically a Range (a span of document content with some extra stuff), so now we select it. We actually only care about the last paragraph, but this is a more direct approach.
Using the Bookmark Range allows the macro to work with derived heading styles as well as the Word standard styles Heading 1 – 9.
Store the desired paragraph style
We want our new paragraph to have a specific style based on the last paragraph of the heading. I like to use the next paragraph style of the last paragraph in the heading. We now have the heading content selected., so we can reference the Paragraphs collection in the Selection object as we've done many times.
In this case, we accessed the Last paragraph of the Paragraphs collection much like we've done for the First paragraph in previous macros.
We store the value in a variable since finding it later after we've moved the insertion point is a little messier.
Insert the new paragraph
Now we can insert the new empty paragraph at the end of the heading range.
We can't use InsertParagraph in this case with selected text, or it will delete our entire heading content!
Move to the new paragraph (Collapsing the Selection)
Then finish the macro like in the other versions. We need to collapse toward the end of the range, so we finish on the new empty paragraph.
Unfortunately, the collapse places us at the beginning of the next heading paragraph, so we need to move back up to our new paragraph.
The default move Unit is by a line, but that is sufficient for our case here.
There are alternative ways than the above steps, but it ends up being a six of one or half a dozen of the other situation. This gets the job done.
Set new paragraph style
Set the new paragraph style if you wish.
Working with the Selection caveat
The above steps are an okay method, but they might cause a little flicker on the screen when we expand and then collapse the selection and move the insertion point.
It's generally a little "messy" to work with the Selection so much since it can result in screen flicker as the macro runs.
We'll talk about disabling screen updates later, but an overall cleaner approach from the beginning would be to define a Range variable and use it to make the changes. However, that is a lesson for another day.
Finish the Macro
Now we just put the commands together.
I assigned this macro to Command+Control+Option+Return in Word for Mac.
I use it mostly in novel notes where I have large outlines where I want to quickly append notes as I’m developing ideas, but I also use it some in my running outline as I write.
Any gotchas?
If the insertion point (not a selection) is at the literal last character of the document, the hidden Word Bookmark won't exist for some reason, and the macro will crash with a mean (okay, informational but still really annoying) error message.
This won't happen often, but if you want to be complete, you could add a check for whether the Bookmark exists.
This is a good practice in general. I just don't want the early macros to look like you have to be an expert to read and write them.
Since we know the specific condition, we could just add a new paragraph at the end of the document, but most of the steps are actually already present for the main macro.
If you're a glutton for the details without further explanation:
But I left it out of the final macro above just for brevity and clarity.